Advanced Tic-Tac-Toe
Project Conducted in the OOP and Data Structure Class
The goal of this project is to develop an Advanced Tic-Tac-Toe game. It is required to design appropriate classes and should use two or more data structures.
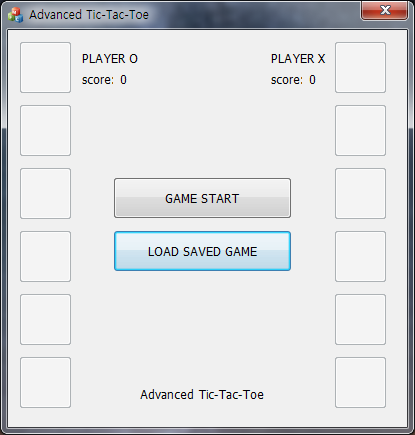
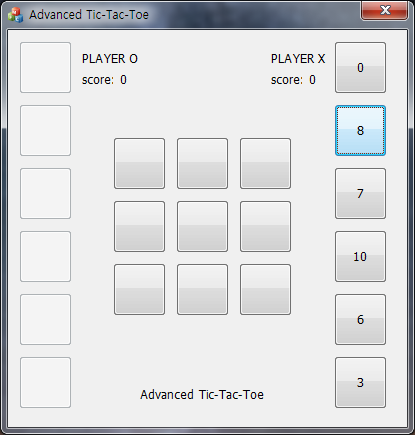
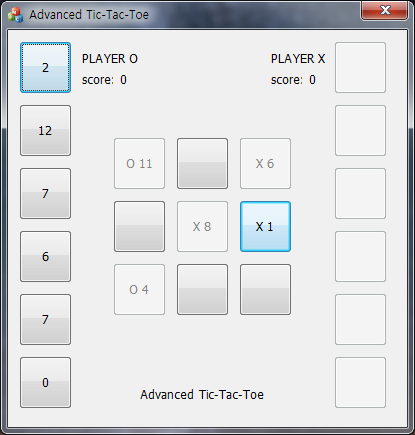
I designed the classes as follows:
Card
holds a shape and number.Player
holds one or moreCard
s.Slot
holds one or moreCard
s and can accept additionalCard
s based on certain conditions.Board
holds one or moreSlot
s and can check if a bingo is made.Dealer
creates uniqueCard
s.Game
initializes other classes and updates the state of the game.
To enable getting a random Card
from Player
, Card
was managed as a Linked-List.
As Board
needs to search for a bingo in a topology composed of Slot
, Slot
were managed as a Graph
Dealer holds randomly generated unique numbers in a Queue.
Since the combination of the shape and number of Card
should be unique and only one instance should exist in a single session of the game,
Dealer
is designed to be the only instance that can instantiate Card
as a std::unique_ptr
.
As an additional feature, I implemented the funtion to save and load the state of the game as JSON. The loading feature includes a logic to verify the validity of the state of the game.